How to Order the WordPress Archive Templates By ACF Date Picker Field
February 7, 2019 By Andrew Gehman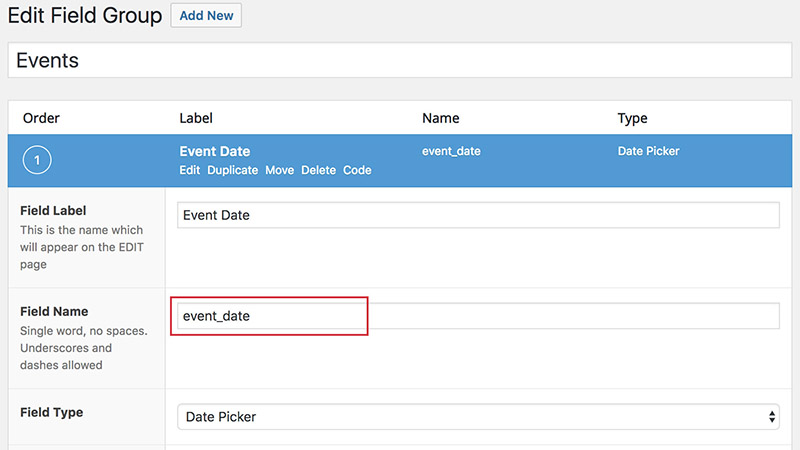
Have you ever needed to adjust the order of posts in a WordPress archive page? By default, WordPress will display posts on archive pages by the date they are published, but ordering by post date isn’t always the best solution. When you need to order posts by something else, here’s how to order the WordPress archive templates by ACF date picker field:
About the ACF Date Picker
Advanced Custom Fields (ACF) is a popular WordPress plugin which allows you to create additional content fields for WordPress posts and pages. The ACF Date Picker field allows users to associate a unique date with a page, post, or custom post type. For example, if you have a custom post type of “event,” you could utilize the ACF Date Picker to create an event date field to store and display a date associated with each event.
How WordPress Archive Pages Work
By default, all WordPress archive pages display posts in reverse chronological order based on the time and date they were published. This means that the most recently published post will appear first and the oldest published post will appear last. If you want to order the posts by a different date, there are a few steps you can take to accomplish it:
How to Order the WordPress Archive Templates by ACF Date Picker Field
1. How to Alter the Query in Functions.php
Let’s assume we have a post type called “event”. For our event post type archive, we want to order by the event date, not the published date of the post. We also want the earliest date to appear first and the latest date appearing last. We can use WordPress Hook pre_get_posts to alter the query before it is run.
function mind_pre_get_posts( $query ) { if( is_admin() ) { return $query; } if( isset($query->query_vars['post_type']) && $query->query_vars['post_type'] == 'event' && $query->is_main_query() ) { $query->set('orderby', 'meta_value'); $query->set('meta_key', 'event_date'); $query->set('order', 'ASC'); } return $query; } add_action('pre_get_posts', 'mind_pre_get_posts');
We don’t want to alter the query in the admin. So, in the code block above, we use the WordPress function is_admin to check if the query occurs in the admin and, if so, to return the query unchanged. The “event” post type archive page is the only place we want to alter the query. This can be done by checking if the post type is set, if the post type is “event”, and if it is in the main query.
If those conditionals are met, the query parameters are changed using $query->set. In order to query by the ACF Date Picker field and display the posts in ascending order, set ‘orderby’ to ‘meta_value’, the ‘order’ to ‘ASC’ and the meta_key to the name of the ACF Date Picker field. (Note: this will also alter the query for any other archive pages for the specific post type, such as taxonomy and date archive pages.)
2. How to Get the Meta Key of the ACF Field
The meta_key will be the field name of your ACF Date Picker field. In our case, the meta key is ‘event_date.’
3. Only Display Future Dates
The query will now display the events custom post type in the desired order. In this specific case, however, we may not want to display past dates. This can be achieved by adjusting our query to pull only dates from today onward. The ACF Date Picker returns dates in the (yyyymmdd) format. By using the PHP Date function, we can compare the value of the current date to the event date.
function mind_pre_get_posts( $query ) { // do not modify queries in the admin if( is_admin() ) { return $query; } // only modify queries for 'events' post type if( isset($query->query_vars['post_type']) && $query->query_vars['post_type'] == 'event' && $query->is_main_query() ) { $query->set('orderby', 'meta_value'); $query->set('order', 'ASC'); $query->set( 'meta_query', array( array( 'key' => 'event_date', 'value' => date('Ymd'), 'compare' => '>=' ))); } // return return $query; } add_action('pre_get_posts', 'mind_pre_get_posts');
Now, we should only see events with an event date of the current date and future dates.
Conclusion
By using the pre_get_posts filter, WordPress developers can alter the order of archive pages. The ACF Date Picker can be a very useful way to order the archive pages in WordPress.
Hi, thanks for the explanation this has done exactly what I wanted by ordering my events by start date and then showing the next upcoming event at the top of my events list by only showing events later than todays date.
The problem i have now is that my older ‘event’ posts are hidden and I would like them to still display as an archive on a different page but with this code the posts with earlier dates are hidden in all archives (as you pointed out) is there a way to allow (or force) the posts to display elsewhere so that they can still be viewed if needed.
Thanks
Sure, there are a few ways to do it. You could alter the conditional statement to only target a specific event archive (add is_post_type_archive(‘event’) for example) and then write a separate conditional to target just the archive that will show everything. Which is essentially what that first code block example will do. You could also write your own query using WP_Query if you’d prefer. You can check out all the WordPress conditionals in the Codex.
Hi,
Is there a way I can do this for a page?
I have elementor pulling in posts from a custom post type and I would like them to be ordered by the date in the ACF fields as opposed to the published date of the article. Elementor allows you to add in a query filter so I have added the one above in and put the code in the functions.php of my child theme but nothing is changing. I think it’s because the code is set for a custom post type?
Thanks
Hi Courtney,
There could be a few things at play in your case. First, I’m not familiar with Elementor so I am not sure how that might effect things. Also, the code block in the examples is only targeting custom post types with the name of ‘event’.
($query->query_vars['post_type'] == 'event')
. You would need to change ‘event’ to the name of the post type is that you are working with. It’s also designed to work with a WordPress archive, which automatically pulls in a defined query, thus the need to alter it with pre_get_posts if you need a different query result. If you are working directly with a page template, I would recommend just writing the query for what you want directly (using WP_Query) in the template rather than trying to filter another query. But again, I’m not sure if that is a capability of Elementor.The codex listed below is successfully working. But I am wondering if there is a way to sort the Event name in alphabetical if two events share the same start date.
Any thoughts?
/* EVENTS LISTED BY CHRONOLOGICAL DATE FILTER */
function event_date() {
if (is_page ( ‘events’ ) ) {
?>
-1,
‘post_type’ => ‘event’,
‘order’ => ‘ASC’,
‘orderby’ => ‘meta_value’,
‘meta_query’ => array(
array(
‘key’ => ‘event_date’,
‘type’ => ‘NUMERIC’, // MySQL needs to treat date meta values as numbers
‘value’ => current_time( ‘Ymd’ ), // Today in ACF datetime format
‘compare’ => ‘>=’, // Greater than or equal to value
),
),
)
);
if( $posts ): ?>
<a href="ID); ?>”>post_title; ?>
ID); ?>
No upcoming events … please check back soon.
<?php
}}
add_action('hook_after_content', 'event_date');
I believe you should be able to do so by passing arrays into the query arguments. https://developer.wordpress.org/reference/classes/wp_query/#order-orderby-parameters
Putting this query in puts my events in the order that I want but if I go to the backend to view one of the older events, I can see it in my list, but once I click view, it pops up not found…Any suggestions?
I would try going to Settings > Permalinks and click Save Changes.
What about grouping events by month?
Example:
August 2021
– Event Title, August 15, 2021
– Event Title 2, August 23, 2021
September 2021
– Event Title, September 2, 2021
Hi,
Thanks so much for this code. It works perfect for the archive page. But unfortunately I’m having some issues with events which have passed the current date. These events give a 404 page. Is there are a way to tweak this code so that older events are still accessible?
Thanks so much!
Try resetting your permalinks. Whenever I see a 404, that’s my first step. Google if you’re not sure how.
OH MY GOD THIS IS SO USEFUL! Thanks for posting this. After Modern Tribes did it’s latest view updates I’ve been trying to figure out how to use ACF as a replacement for it. This is SUPER helpful and will save me a bunch of time.
FYI, if anyone is trying to query a sub field of a group, all you need to is prepend the name of the group field, and then add an underscore before the subfield name. Here’s a link that explains more.
https://support.advancedcustomfields.com/forums/topic/meta_query-for-a-group-field-sub-field/