How to Enhance Critical Inline CSS Using Cookies in WordPress
May 14, 2020 By Hiep Dinh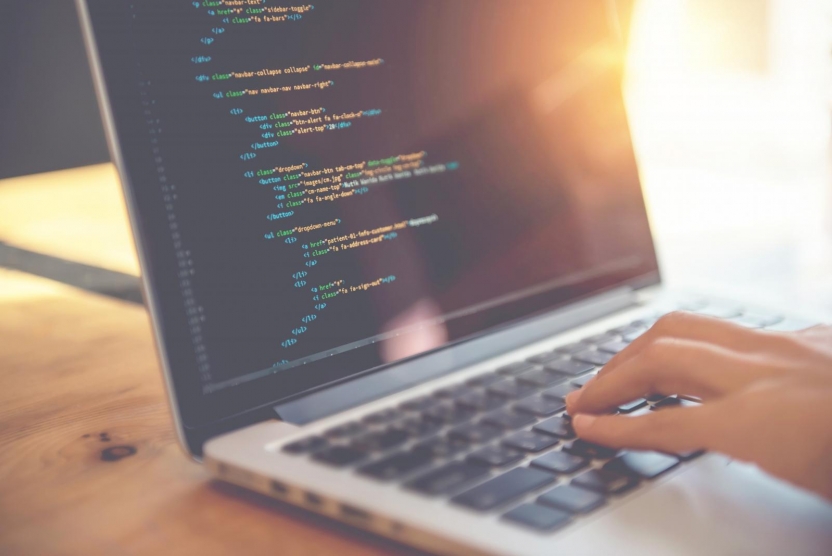
There are a couple of options for creating critical CSS in WordPress in order to improve website performance. And, once your inline CSS loads in the <head>, the full style sheet will load asynchronously shortly afterward. Then, it’s typically stored in your browser’s cache. This is a good start towards better page speeds. But, you can improve your performance by serving the inline CSS on the first visit only, and then serving the browser’s full, cached style sheet for subsequent site visits while omitting the inline CSS. This will save page weight, which can further improve your website’s performance. To do this, you’ll need to know how to enhance critical inline CSS using cookies in WordPress. Here are the steps you need to set up the cookie and handle the related logic for CSS loading via functions.php:
Step 1: Set Up a Cookie in functions.php
The following code uses PHP’s setcookie() function to set up the cookie. We’ll use this cookie to determine which version of the style sheet to serve – the inline CSS or the cached style sheet. The setcookie() function has the following parameters:
- Cookie name
- Cookie value
- Expire time
- Path
- Domain
- Secure
- httponly
In this example, we’ll name the cookie “full-css” and we’ll set its expire time to 30 days. It should look something like this:
add_action('init', 'full_css'); function full_css() { setcookie('full-css', true, time() + (60 * 60 * 24 * 30), '/'); }
Step 2: Set Up a Function for Inserting the Critical CSS in the Header
You can use the $_COOKIE[] variable to call the cookie you created and test it to determine if this is the user’s first visit.
When it’s Not the User’s First Visit
We’ll serve the full style sheet when it’s not the user’s first visit. That function should look something like this:
if(!function_exists('put_scripts_in_header')) { function put_scripts_in_header() { // link to full css $allcss = get_template_directory_uri() . '/scss/base.css'; // if the cookie is set, then this is not the first visit, so serve the full stylesheet if( isset($_COOKIE['full-css']) ) { wp_enqueue_style( 'full', $allcss, array(), '1.0.0'); }
When it is the User’s First Visit
If this is the user’s first visit, we’ll put the inline critical styles in the header and then load the rest of the style sheet afterward, asynchronously. The conditional from lines 6 to 18 are based on a scenario where the inline critical CSS is different for the home page and the blog page, with a fallback to a generic “inline.css” for all the other pages:
// else show critical CSS else { echo '<style>'; if( is_front_page() ) { include get_template_directory() . '/scss/inline/home.css'; } elseif( is_home() ) { include get_template_directory() . '/scss/inline/blog.css'; } else { include get_template_directory() . '/scss/inline/inline.css'; } echo '</style>'; echo '<link rel="preload" href="' . $allcss . '" as="style">'; echo '<link rel="stylesheet" href="' . $allcss . '" media="print" onload="this.media=\'all\'">'; echo '<noscript>'; echo '<link rel="stylesheet" href="' . $allcss . '" />'; echo '</noscript>'; }
On lines 22 and 23 we’re using a technique described by The Filmament Group to asynchronously load the full style sheet (we previously used loadCSS), and on lines 25 to 27 there is a <noscript> fallback to allow the style sheet to load if JavaScript is disabled.
The Full Code
Once you have all the pieces put together, the full code to enhance critical inline CSS using cookies in WordPress should look something like this:
if(!function_exists('put_scripts_in_header')) { function put_scripts_in_header() { // link to full css $allcss = get_template_directory_uri() . '/scss/base.css'; // if not visiting the first time, show the full css if( isset($_COOKIE['full-css']) ) { wp_enqueue_style( 'full', $allcss, array(), '1.0.0'); // else show critical css } else { echo '<style>'; if( is_front_page() ) { include get_template_directory() . '/scss/inline/home.css'; } elseif( is_home() ) { include get_template_directory() . '/scss/inline/blog.css'; } else { include get_template_directory() . '/scss/inline/inline.css'; } echo '</style>'; echo '<link rel="preload" href="' . $allcss . '" as="style">'; echo '<link rel="stylesheet" href="' . $allcss . '" media="print" onload="this.media=\'all\'">'; echo '<noscript>'; echo '<link rel="stylesheet" href="' . $allcss . '" />'; echo '</noscript>'; } } } add_action('wp_enqueue_scripts', 'put_scripts_in_header');
A Small, But Worthwhile, Improvement
The path to better site performance largely involves a series of little enhancements: optimizing images, leveraging caching, adding critical inline CSS, etc. Since the critical inline CSS can’t be cached, your pages will be a little bit heavier with that extra code sitting in the <head>. This extra bulk has no purpose when the browser already has a cached copy of the style sheet after the first visit.
Serving that cached style sheet conditionally using cookies is yet another small enhancement that should result in better page speeds and a better experience for your users! And, since page speed and SEO are connected, making these enhancements and improving your website’s performance can also help you get more traffic too!
View Comments