How to Display Recent Blog Posts on the Homepage
June 30, 2017 By Andrew Gehman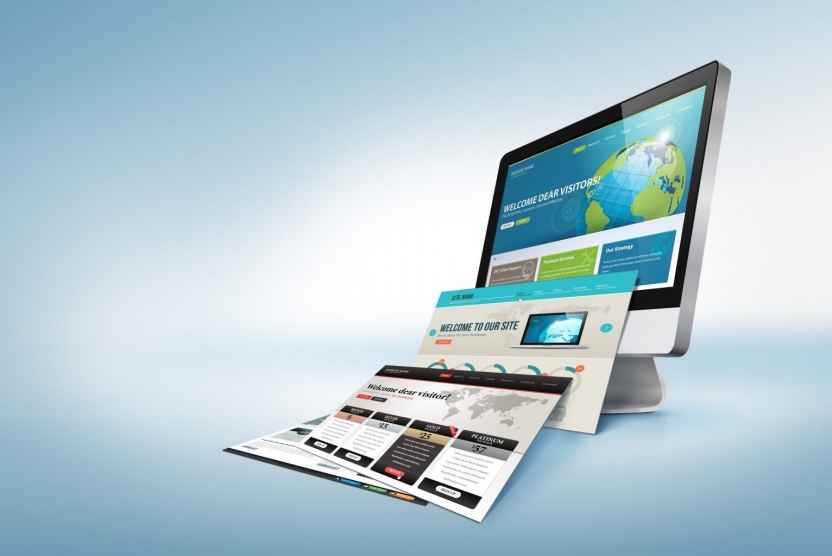
One of the most common client requests is to display the most current blog posts on their front page. So, for this tutorial, we will go over how to take the four most recent blog posts and display them horizontally across the front page. We’ll also be using a static front page, and working in the “front-page.php” page template.
Define CSS
To begin, I will define some CSS
.col-1-4 {
width: 25%;
float: left;
padding: 10px;
}
.post-wrap {
padding: 20px;
}
Create the HTML
And create the HTML we’ll be using below. This should be placed in the preferred location: the front-page.php template.
<div class=”wrap”>
<div class=”col-1-4 fp-posts”>
<div class=”post-wrap”>
<div class=”post-content”>
</div>
</div>
</div>
<div class=”col-1-4 fp-posts”>
<div class=”post-wrap”>
<div class=”post-content”>
</div>
</div>
</div>
<div class=”col-1-4 fp-posts”>
<div class=”post-wrap”>
<div class=”post-content”>
</div>
</div>
</div>
<div class=”col-1-4 fp-posts”>
<div class=”post-wrap”>
<div class=”post-content”>
</div>
</div>
</div>
</div>
Write it Once
Since we will be using PHP to dynamically output our content, we won’t need to code out the ‘div.col-1-4.fp-posts’ all four times. We only need to write it once.
<div class=”wrap”>
<div class=”col-1-4 fp-posts”>
<div class=”post-wrap”>
<div class=”post-content”>
</div>
</div>
</div>
</div>
Featured Images (‘Post Thumbnails’)
As we did in my previous post, Set the Featured Image as a Background Image in WordPress, we’re going to assume that featured images (or ‘post thumbnails’) are enabled in the WordPress theme. If this feature is not already enabled, we’ll want to include add_theme_support( ‘post-thumbnails’ ); in the functions.php file.
Query the Database
Our next steps is to query the database using the WordPress’ WP Query class to retrieve the blog posts that we wish to display on our front page.
We begin this process by creating a new WP_Query object, and saving it in a variable called $query.
<?php $query = new WP_Query( $query_args ); ?>
WP_Query can accept an array of parameters to filter the results returned by the query. So, we’ll create an array call $query_args and define the parameters we’d like to pass into the WP_Query object. We know that we want to return posts, and we know that we only want to return the four most recent posts. To do so, we will define the ‘post type’ as ‘post’ and setting the ‘posts_per_page’ to 4. Since we want the four most recent blog posts, I’ve set the ‘orderby’ to ‘date’ and ‘order’ to ‘DESC’, which will display the results in descending order (which in this case is newest to oldest by date). These parameters are both defaults, so we would actually get the desired result even if we omitted this parameter. If you wanted the oldest four posts, you would set the order to ‘ASC’.
<?php $query_args = array(
‘post_type’ => ‘post’,
‘posts_per_page’ => 4 ,
‘orderby’ => ‘date’,
‘order’ => ‘DESC’
);
$query = new WP_Query( $query_args ); ?>
Iterate and Display
Now that we’ve set up our query, we’re going to use the WordPress Loop to iterate through our results and display them on the page. The primary difference between our custom loop and a normal WordPress loop is that we will need to reference our new WP_Query object rather than use the default loop.. For example, instead of ‘if ( have_posts() )’, we will use ‘if ( $query->have_posts() )’.
<?php if ( $query->have_posts() ) : ?>
<?php while ( $query->have_posts() ) : $query->the_post(); ?>
<!– do stuff … –>
<?php endwhile; ?>
<?php endif; ?>
Integrate HTML
Next, we need to integrate our HTML along the PHP functions to output our content. We have a ‘div.wrap’ as a parent element “wrapping” our content.
The ‘div.post-wrap’ will be the element that defines the post thumbnail as a background image. (See my earlier blog post for more in depth details about setting the featured image as a background image.)
In short, we’ll be using wp_get_attachment_image_src to retrieve the featured image and store it in a variable called $backgroundImg. Next, we’ll echo that variable out as inline CSS in our code.
I’ve also used ‘background-repeat: no-repeat’, and ‘background-size: cover’ to style the background image to my liking. You may want to use some of the other CSS background properties to best style your background image.
<?php $query_args = array(
‘post_type’ => ‘post’,
‘posts_per_page’ => 4 ,
‘order’ => ‘DESC’
);
$query = new WP_Query( $query_args ); ?>
<?php if ( $query->have_posts() ) : ?>
<div class=”wrap”>
<?php while ( $query->have_posts() ) : $query->the_post(); ?>
<?php $backgroundImg = wp_get_attachment_image_src( get_post_thumbnail_id($post->ID), ‘full’ );?>
<div class=”col-1-4 fp-posts”>
<div class=”post-wrap” style=”background: url(‘<?php echo $backgroundImg[0]; ?>’) no-repeat; background-size: cover;”>
<div class=”post-content”>
</div>
</div>
</div>
<?php endwhile; ?>
</div> <!– .wrap –>
<?php endif; ?>
<?php wp_reset_postdata(); ?>
Finishing Touches
Now for the finishing touches. We’ll start by wrapping the ‘div.post’ in an anchor tag, and then link to the original post using WordPress’ the_permalink function.
<a href=”<?php the_permalink(); ?>”>
<div class=”post-wrap” style=”background: url(‘<?php echo $backgroundImg[0]; ?>’) no-repeat; background-size: cover;”>
<div class=”post-content”>
</div>
</div>
</a>
Display Title and Content
At this point we just need to display the title and content:
<?php $query_args = array(
‘post_type’ => ‘post’,
‘posts_per_page’ => 4 ,
‘order’ => ‘DESC’
);
$query = new WP_Query( $query_args ); ?>
<?php if ( $query->have_posts() ) : ?>
<div class=”wrap”>
<?php while ( $query->have_posts() ) : $query->the_post(); ?>
<?php $backgroundImg = wp_get_attachment_image_src( get_post_thumbnail_id($post->ID), ‘full’ );?>
<div class=”col-1-4 fp-posts”>
<a href=”<?php the_permalink(); ?>”>
<div class=”post-wrap” style=”background: url(‘<?php echo $backgroundImg[0]; ?>’) no-repeat; background-size: cover;”>
<div class=”post-content”>
<h3><?php the_title(); ?></h3>
<?php the_content(); ?>
</div>
</div>
</a>
</div>
<?php endwhile; ?>
</div> <!– .wrap –>
<?php endif; ?>
<?php wp_reset_postdata(); ?>
Display an Excerpt
Perhaps you do not want to display the entire blog post on the page, but rather just a snippet (this is recommended to avoid SEO duplicate content issues). If so, you can use WordPress’ the_excerpt function in place of the the_content.
You may also notice that I’ve placed ‘wp_reset_postdata‘ function at the end of our code block. This may be needed to reset the global $post variable in the event you need to refer to the original query.
Now that we’re finished, our set of 4 blog posts on the front page will look something like this:
Wrapping Up
WP_Query is very powerful and useful feature of WordPress with numerous parameters that you can use to customize your queries. For more details, check out the WP_Query entry in the WordPress Codex.
Can I use the same method on a website I developed (not powered by WordPress)
You could use the same concepts, but the example code includes WordPress functions, etc that won’t work outside of WordPress.
How can I switch from recent posts to more popular posts?
Hi that is great. Though I’m using elementor page builder and it’s easy. I have a question if you can be so kind to advise. Is there any good impact on the seo if blog posts are placed on homepage? Will it help in ranking? I mean is good or bad or no impact at all? Please guide
Thank you
In general, featuring your recent posts on the homepage is a good move. If you’re investing in SEO, that means your blog content should be valuable and helpful to people who would be visiting your website. Featuring blog content on the homepage can be a good way to get more views on that content. At the same time, it provides a pathway for users that are still just looking, researching, and gathering information. All of this works together to get more eyes on your content, more engagement on your website, and could result in someone who finds your content sharing it on social media or even linking to it from their own blog or website. In this way, featuring recent blog posts on the homepage can have a positive impact that may help increase your site’s overall authority and relevancy, which can give it a ranking boost, and also helps support your other SEO efforts. At the same time, it can also result in more people signing up for your newsletter (if you have one), submitting a contact form, or calling you.
Hello how can i display only blog from a certain category, or tag?
You would add an argument to your query for either the category id or slug or the tag id or slug.
https://developer.wordpress.org/reference/classes/wp_query/
thank you for replying. Your guide is really helpful. 🙂
I tried to place this code in my website but I got error 500. Then I checked the code on phpcodechecker.com and there it shows the following error:
Parse error: syntax error, unexpected end of file, expecting elseif (T_ELSEIF) or else (T_ELSE) or endif (T_ENDIF) in your code on line 27.
What could be the reason for this?
Sorry, it looks like I was missing an endif at the end of my code block. I’ve updated the code.