How to Randomize ACF WordPress Plugin’s Repeater Fields
November 22, 2018 By Andrew Gehman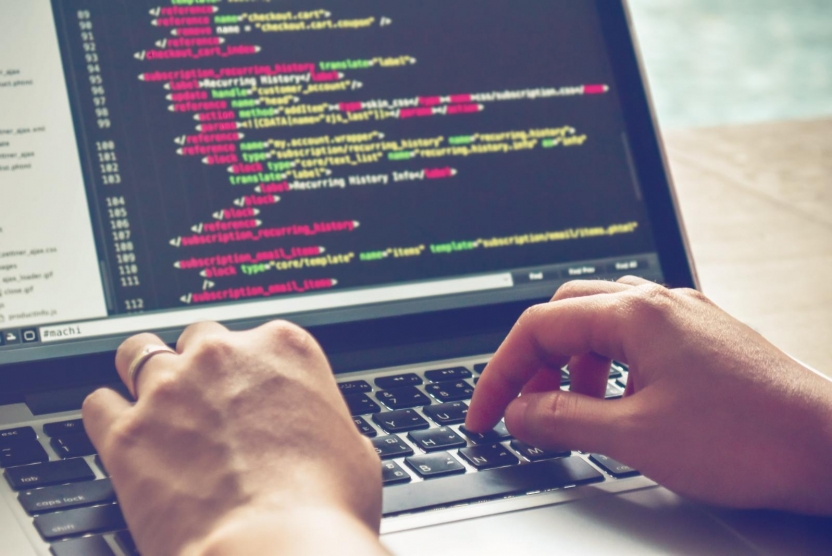
Advanced Custom Fields is one of the most popular and useful WordPress plugins. Perhaps the most popular feature of the pro version of ACF, is the repeater field. The ACF Repeater field allows you to create unlimited repeating sub fields. Occasionally, we have a need to randomize the order in which these repeater sub fields are output. Here are three examples of how to randomize ACF WordPress plugin’s repeater fields that will randomly grab one, some, or all of the items in an ACF repeater.
If you’ve used an ACF Repeater before, you’re likely familiar with the standard way to loop through a repeater using the have_rows and the_row functions. I’ll use a simple PHP for loop to achieve the same result. We begin all of these examples by using the get_field function and the field name of the repeater field to get the contents of the repeater field. We then use the PHP is_array function to ensure we aren’t dealing with an empty array.
How to Randomly Display All Items In a Repeater Field Using PHP Shuffle
PHP’s shuffle randomizes the order of the elements in an array. After we run the shuffle function on our array, we can loop through the array and echo out the now randomized sub fields.
<?php $random_cars = get_field( 'car_types' ); ?> <?php if ( is_array( $random_cars ) ) { ?> <?php shuffle( $random_cars ); ?> <?php foreach ($random_cars as $random_car ) { ?> <p><?php echo $random_car['car_model']; ?></p> <?php } ?> <?php } ?>
How to Randomly Display a Certain Number of Items in a Repeater Field
PHP’s array_rand takes an array and returns one or more randomized keys from an array. We need to pass our repeater field array and the number of random keys we need to return to array_rand, and then loop through those results by referencing the original array, the randomly returned keys, and the ACF sub field.
Note: Be aware that if you try to return more items than there are in the array, you will get an error. So, you may want to check the amount of items in the array by using the PHP count function, and if it’s less than or equal to the number of random items to return, set up a conditional to use shuffle instead.
<?php $all_cars = get_field( 'car_types' ); ?> <?php if ( is_array( $all_cars ) ) { ?> <?php $random_cars = array_rand( $all_cars, 3 ); ?> <?php foreach ($random_cars as $random_car ) { ?> <p><?php echo $all_cars[$random_car]['car_model']; ?></p> <?php } ?> <?php } ?>
How to Randomly Display One Item From a Repeater Field
To grab one random item from our array, we’ll use array_rand again and simply leave out the 2nd parameter that defines how many items should be picked. This will result in just one random key being returned. We can then work with that item by referencing our array with the random key and the name or the ACF sub field.
<?php $cars = get_field( 'car_types' ); ?> <?php if( is_array( $cars ) ) { ?> <?php $car = array_rand( $cars ); ?> <?php echo $cars[$car]['car_model'];?> <?php } ?>
These a few pretty useful methods to get random items from an ACF Repeater field. There are a lot of ways you can customize existing plugins; you can also create your own. If you have an idea for a WordPress plugin, take a look at our quick overview of how to get started with creating a WordPress plugin.
Wow. Thank you for posting this! It was exactly what I needed to create a randomized Amazon ad block with ACF and couldn’t find the answer anywhere else.