How to Link an Anchor Tag to a Target Location on a Different Page with Expanded Content and a Fixed Header
January 31, 2017 By Andrew Gehman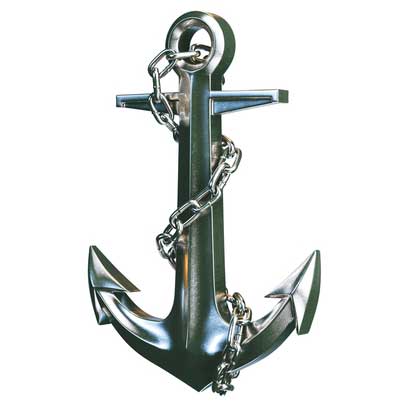
In this blog post, we’re going to explore how to link an anchor tag to a target location on another page. Plus, we’re going to take it a few steps further and explore how we handle linking to those targets on a page with a fixed header, and how to automatically display content that exists within expanding content.
Linking to a specific spot on a page is pretty simple. You give the targeted content an id.
<div id="inpage">Here is the location on the page to where you want to link.</div>
And then create your link. Rather than assigning a URL to the href attribute, you assign it a URL fragment (a hashtag followed by the id of the linked content.
<a href="#inpage">Link to a spot within the page</a>
If you wish to link to an in page content section on a different page, it’s just a matter of adding that URL before the URL fragment. Again, this is a pretty simple concept.
<a href="#http://www.domain.com/#inpage">Link to a spot within the content on a different page.</a>
Fixed Header Problem
Now, what if you are dealing with a fixed header? Your link doesn’t know that you have a fixed header defined in your CSS, and since fixed content is taken out of the normal flow of the DOM, it won’t take the hight of that fixed content into consideration. What results, sadly, is that some or all of the linked content is likely to be hidden behind the fixed header.
Fixed Header Solution
If you are able to add a class to your targets, you can reference that class…
<div id="inpage" class="linktarget">Here is the location on the page to where you want to link.</div>
… in your CSS with a before pseudo element to offset the height of the header. You just need to adjust the height to match that of your header (or more specifically, the height of the fixed content at the top of the page.
.linktarget:before { content:""; display:block; height:120px; /* the height of the fixed header*/ margin:-120px 0 0; /* the negative of the fixed header height */ }
If adding a class is not an option, you can do the same with the target pseudo element and a before pseudo element.
:target:before { content:""; display:block; height:120px; /* the height of the fixed header*/ margin:-120px 0 0; /* the negative of the fixed header height */ }
Of course, the height of your header will likely be different, and the height of the header may change depending on screen size, so it may require you to test and adjust this height at a variety of break points.
Expanded Content Problem
In some cases, you may need to target in-page content that is ‘hidden’ by default and only revealed when a button or other element is ‘clicked,’ such as JavaScript accordion style layout. (See the basic example from the CSS Tricks team on CodePen)
When directing the user to another page, you may not want to subject them to scanning over a list of titles looking for the desired content, especially if they just clicked on a link expecting to be taken directly to specific content.
So, how do we take the user directly to this content and have the content open automatically?
Expanded Content Solution
This next bit of magic we will make happen with JavaScript and the jQuery library. But first, let’s take a quick look at the HTML.
<div id="one" class="clicker"> <h3>Click Here</h3> </div> <div class="clicker-content"> Sale partem euismod ea pro. Laboramus moderatius nam ei. Omittam antiopam nam ei, nam laudem salutandi ne. Id possim deserunt deseruisse his, duo sonet dissentias persequeris ad, cu quod porro vivendum sit. Qui ne utroque nonumes, sit cu modus aperiri tincidunt, mei inani vivendum cu. His te quot nihil sententiae, eos copiosae ponderum ut, ne porro recteque inciderint has. Adipisci recteque ei pri. Ne pro tollit volumus, quo et quidam viderer. Melius vocibus id ius. Falli tibique usu cu, alia sale vis ei. Ex quo nibh minimum, ius cu vidit omittantur signiferumque. </div> <div id="two" class="clicker"> <h3>Click Here</h3> </div> <div class="clicker-content"> Sale partem euismod ea pro. Laboramus moderatius nam ei. Omittam antiopam nam ei, nam laudem salutandi ne. Id possim deserunt deseruisse his, duo sonet dissentias persequeris ad, cu quod porro vivendum sit. Qui ne utroque nonumes, sit cu modus aperiri tincidunt, mei inani vivendum cu. His te quot nihil sententiae, eos copiosae ponderum ut, ne porro recteque inciderint has. Adipisci recteque ei pri. Ne pro tollit volumus, quo et quidam viderer. Melius vocibus id ius. Falli tibique usu cu, alia sale vis ei. Ex quo nibh minimum, ius cu vidit omittantur signiferumque. </div> <div id="three" class="clicker"> <h3>Click Here</h3> </div> <div class="clicker-content"> Sale partem euismod ea pro. Laboramus moderatius nam ei. Omittam antiopam nam ei, nam laudem salutandi ne. Id possim deserunt deseruisse his, duo sonet dissentias persequeris ad, cu quod porro vivendum sit. Qui ne utroque nonumes, sit cu modus aperiri tincidunt, mei inani vivendum cu. His te quot nihil sententiae, eos copiosae ponderum ut, ne porro recteque inciderint has. Adipisci recteque ei pri. Ne pro tollit volumus, quo et quidam viderer. Melius vocibus id ius. Falli tibique usu cu, alia sale vis ei. Ex quo nibh minimum, ius cu vidit omittantur signiferumque. </div>
A Trio of Divs
We have a trio of div’s, each with their own id, and a class name of ‘clicker.’ Below each of those divs, we have divs with the class name of ‘clicker-content.’
The ‘clicker-content’ divs are hidden by default and only displayed on click with a little bit of jQuery (as seen below).
$('.clicker').on('click', function(){ $(this).next('.clicker-content').slideToggle(); });
This works fine when you are already on the page and you’re manually clicking, and we have ID’s so we can link directly to them in-page. Now we need to make the correct in page content open automatically when we arrive via a link with a matching hash from another page.
To accomplish this, we’ll use JavaScript’s window.location.hash. This will return the ‘hash’ from the url. So, if the url is www.domain.com/page/#two, it’ll return ‘#two.’
Next we’ll use a conditional to check if we have a url with a hash, and if we do, we’ll set the hash to a variable. That hash will be the same as the ID of the element on which we wish perform the action (in this case, clicking to perform the slideToggle), So, we’ll assign the returned hash to a jQuery selector and use the jQuery click method to perform the mouse action of clicking.
if(window.location.hash) { var hash = window.location.hash; $(hash).click(); }
Finally, we’ll also wrap out code in a document.ready function to ensure page is loaded before attempt to run any of our code. So, the final Javascript will look like this:
$(document).ready(function(){ $('.clicker').on('click', function(){ $(this).next('.clicker-content').slideToggle(); }); if(window.location.hash) { var hash = window.location.hash; $(hash).click(); } });
And when it’s all finished, it should function something like this…
Boom. A seemingly complex issue, handled with just a bit of CSS and Javascript.
View Comments